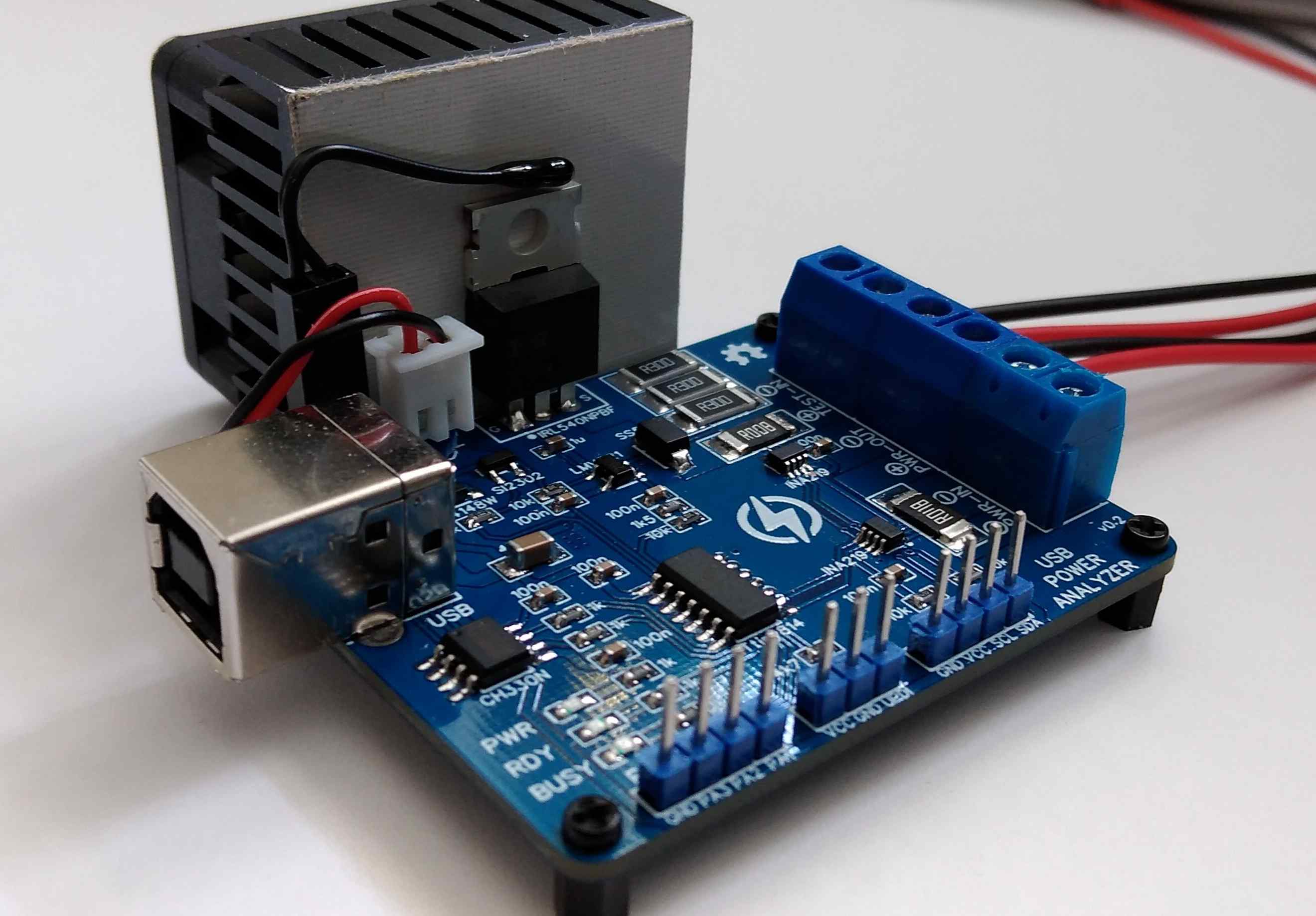
ATtiny814 Power Analyzer
STDATtiny814 Power Analyzer
8.4k
0
0
2
Mode:Full
License
:CC-BY-SA 3.0
Creation time:2020-03-14 19:14:53Update time:2022-05-10 05:47:30
Description
Design Drawing
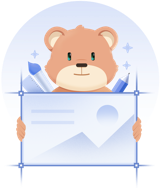
BOM
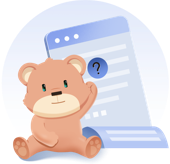

Add to Album
0
0
Share
Report
Project Members
Followers0|Likes0
Related projects
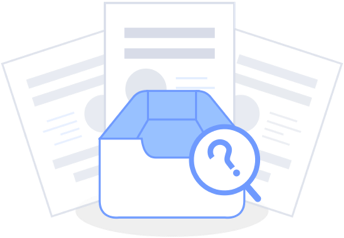
Comment