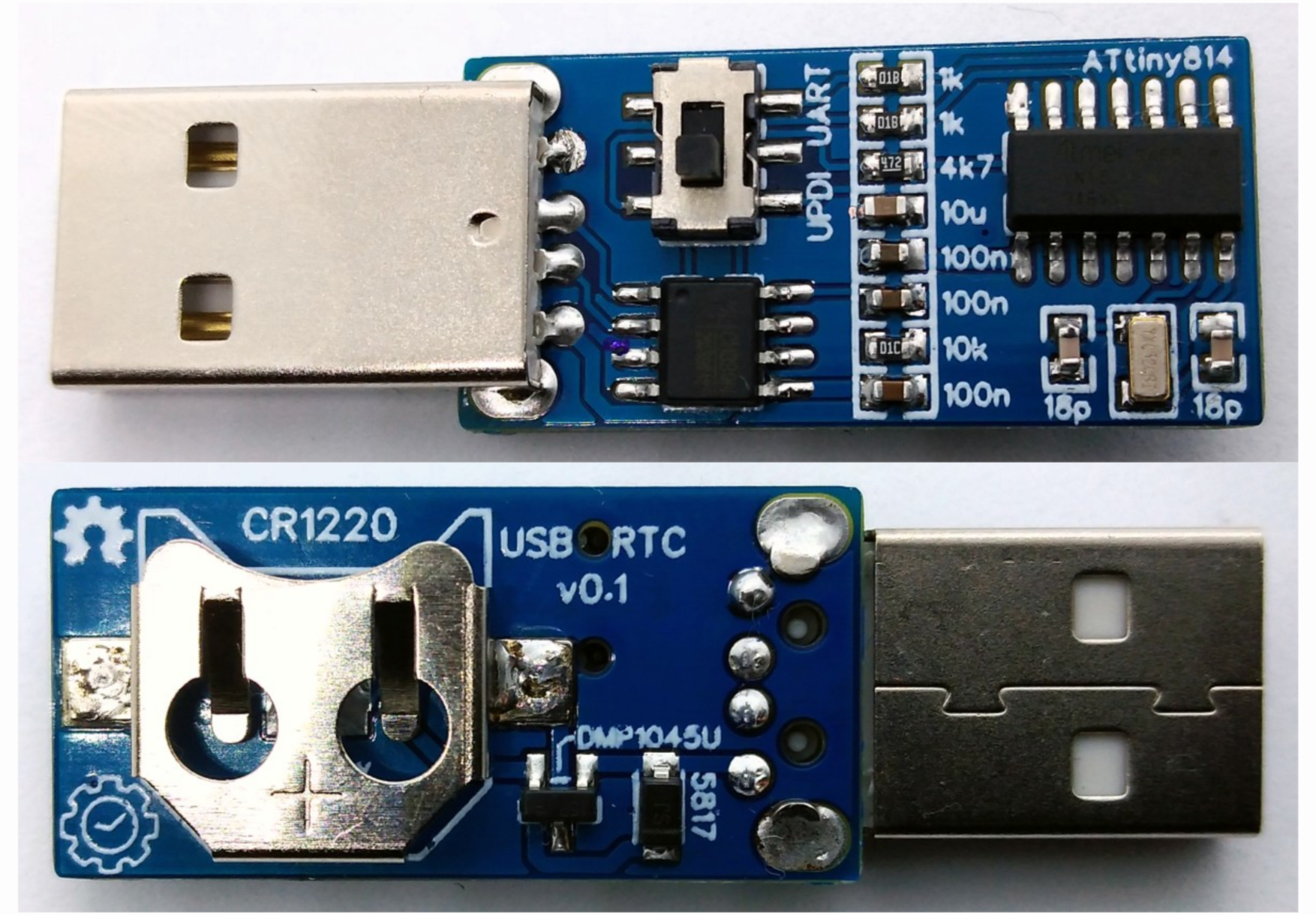
ATtiny814 USB-RTC
STDATtiny814 USB-RTC
2.6k
0
0
0
Mode:Full
License
:CC-BY-SA 3.0
Creation time:2021-06-29 18:00:14Update time:2021-12-22 17:00:29
Description
Design Drawing
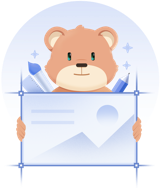
BOM
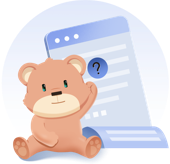

Add to Album
0
0
Share
Report
Project Members
Followers0|Likes0
Related projects
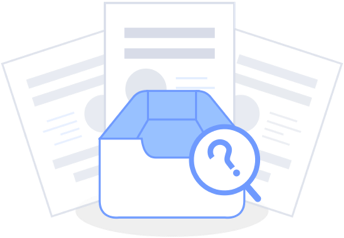
Comment