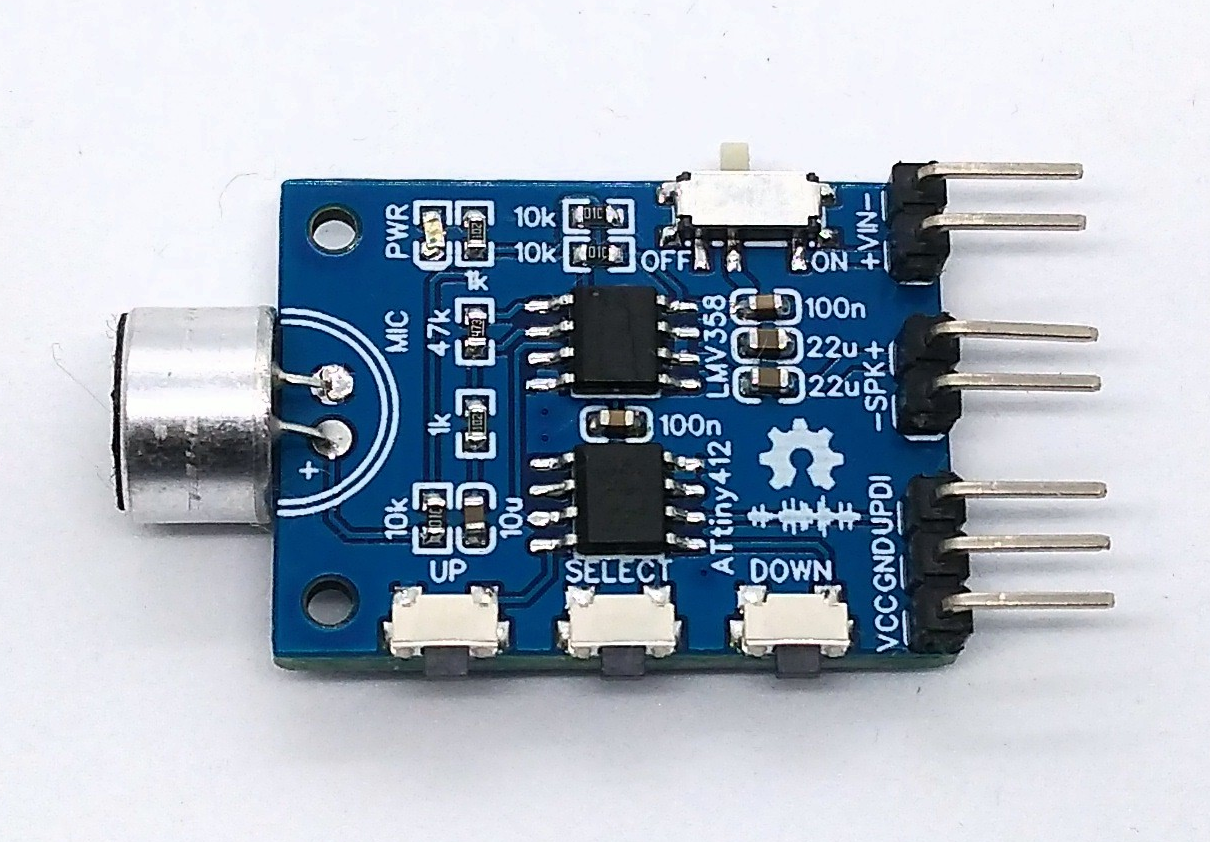
ATtiny412 Voice Changer
STDATtiny412 Voice Changer
1.3k
0
0
0
Mode:Full
License
:CC-BY-SA 3.0
Creation time:2022-06-06 09:55:04Update time:2022-06-29 17:10:35
Description
Design Drawing
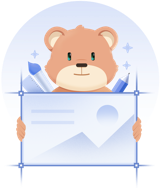
BOM
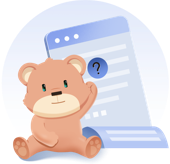

Add to Album
0
0
Share
Report
Project Members
Followers0|Likes0
Related projects
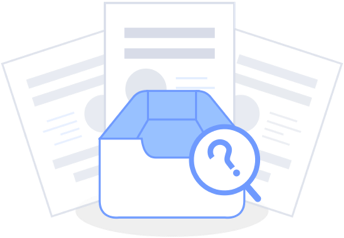
Comment