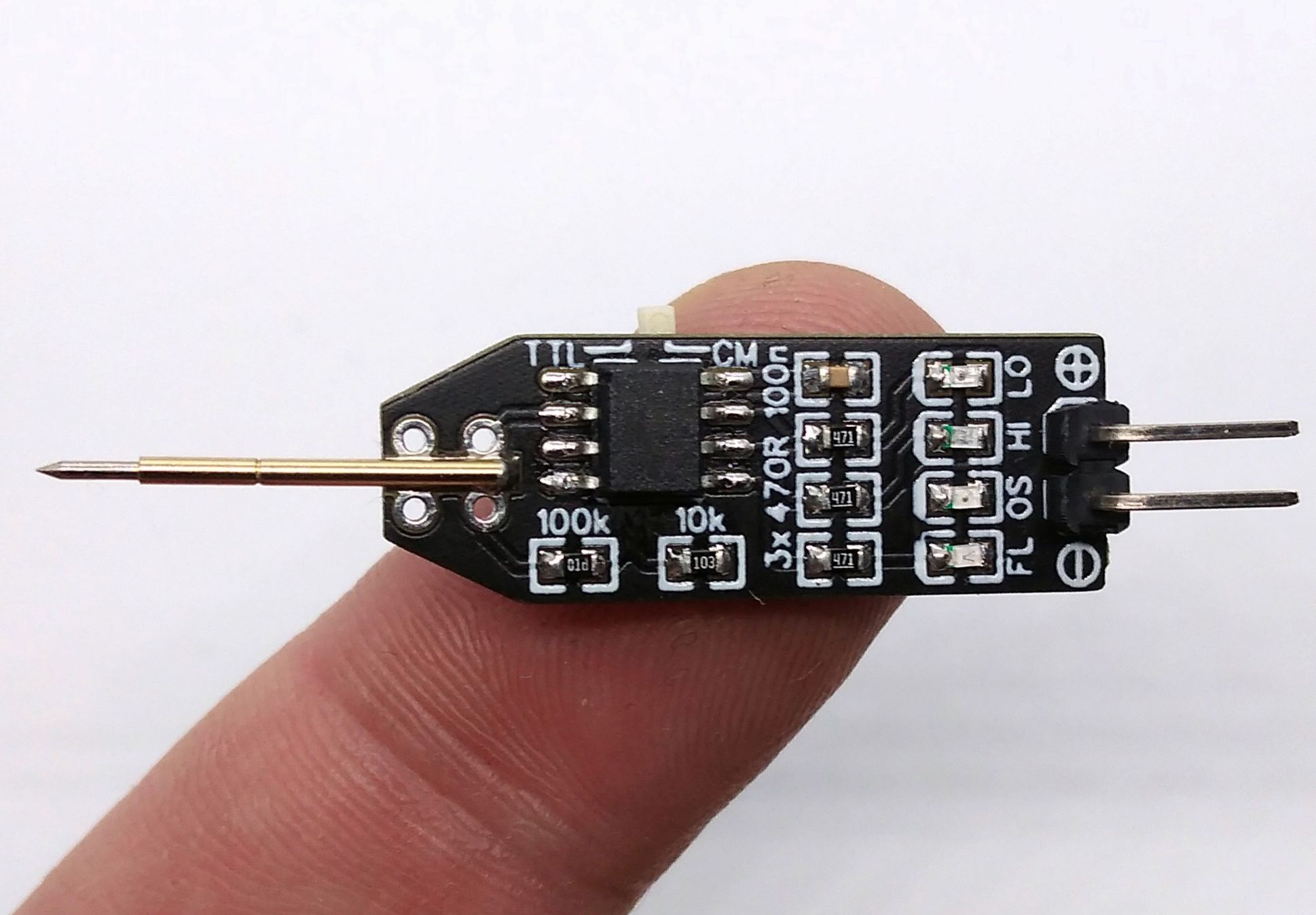
ATtiny13 TinyProbe
STDATtiny13 TinyProbe
31k
0
0
3
Mode:Full
License
:CC-BY-SA 3.0
Creation time:2020-08-22 10:02:46Update time:2022-06-29 17:29:08
Description
Design Drawing
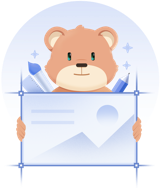
BOM
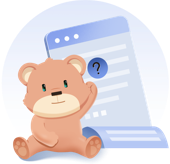

Add to Album
0
0
Share
Report
Project Members
Followers0|Likes0
Related projects
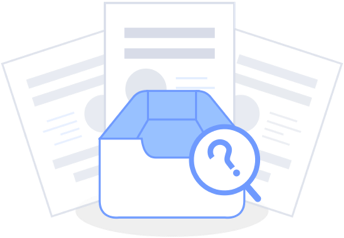
Comment