Your EasyEDA usage duration is brief. In order to avoid advertising information, this action is not supported at present. Please extend your EasyEDA usage duration and try again.
Editor Version×
Standard
1.Easy to use and quick to get started
2.The process supports design scales of 300 devices or 1000 pads
3.Supports simple circuit simulation
4.For students, teachers, creators
Profession
1.Brand new interactions and interfaces
2.Smooth support for design sizes of over 5,000 devices or 10,000 pads
3.More rigorous design constraints, more standardized processes
# NeoController - NeoPixel Controller and Tester based on ATtiny13A
An ATtiny13 is more than sufficient to control almost any number of NeoPixels via an IR remote. The NeoController was originally developed as a tester for 800kHz NeoPixel strips. Since there was still so much flash left in the ATtiny13, an IR receiver was integrated so that some parameters can be controlled with an IR remote control. In this way, it is also suitable as a simple and cheap remote-controlled control unit for NeoPixels. Due to its small size (21.6mm x 11.4mm), it can be soldered directly to the LED strip without any problems. The power supply via a USB-C connection enables currents of up to 3A. There is still more than a third of the flash memory left for additional ideas.
- Project Video (YouTube) : https://youtu.be/HLtST_1GFfo
- Firmware (Github): https://github.com/wagiminator/ATtiny13-NeoController
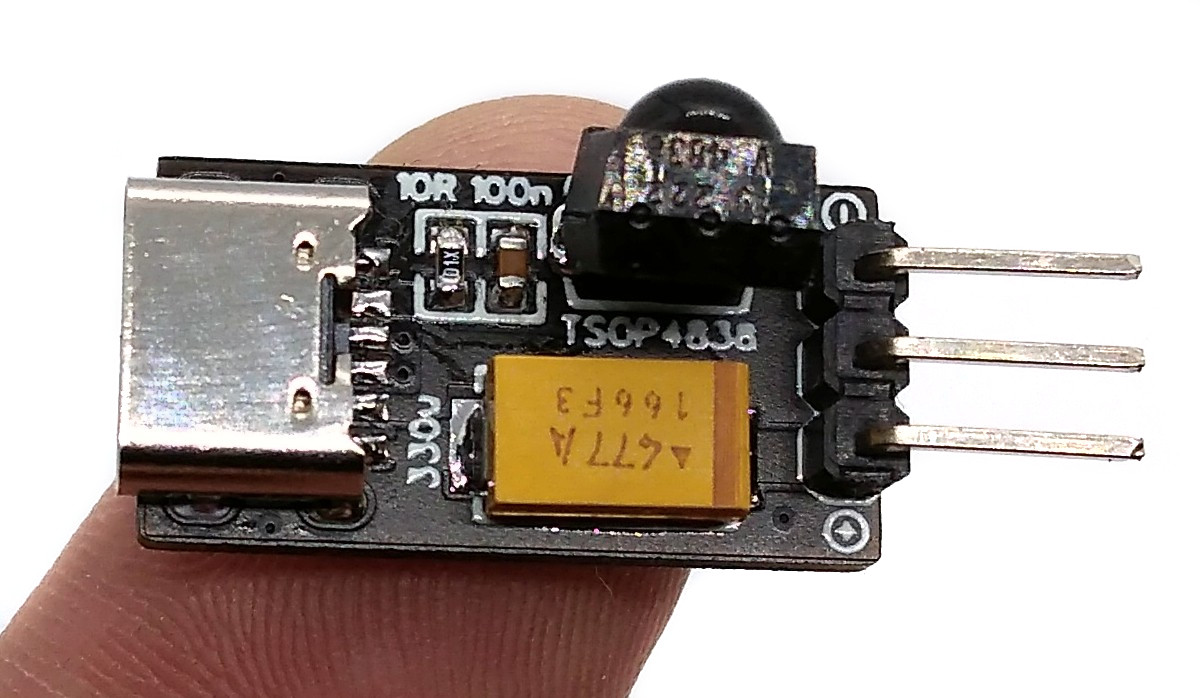
# Hardware
The wiring is pretty simple. For a breadboard test you can use the following simplified schematic:
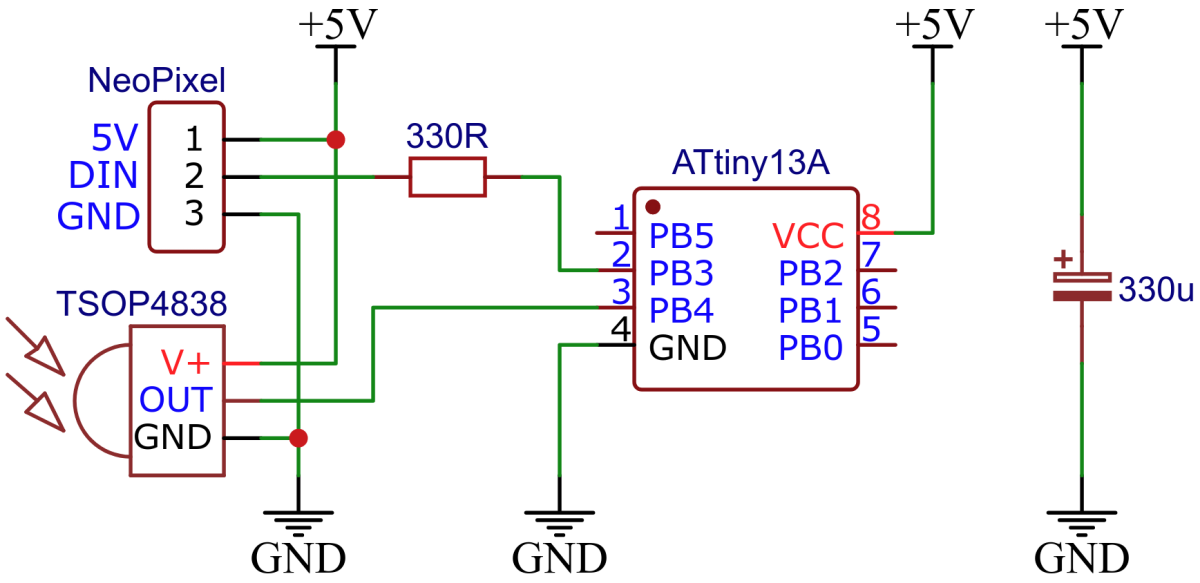
# Software
## NeoPixel Implementation
The control of NeoPixels with 8-bit microcontrollers is usually done with software bit-banging. However, this is particularly difficult at low clock rates due to the relatively high data rate of the protocol and the strict timing requirements. The essential protocol parameters for controlling the WS2812 NeoPixels (or similar 800kHz addressable LEDs) can be found in the [datasheet](https://cdn-shop.adafruit.com/datasheets/WS2812.pdf).
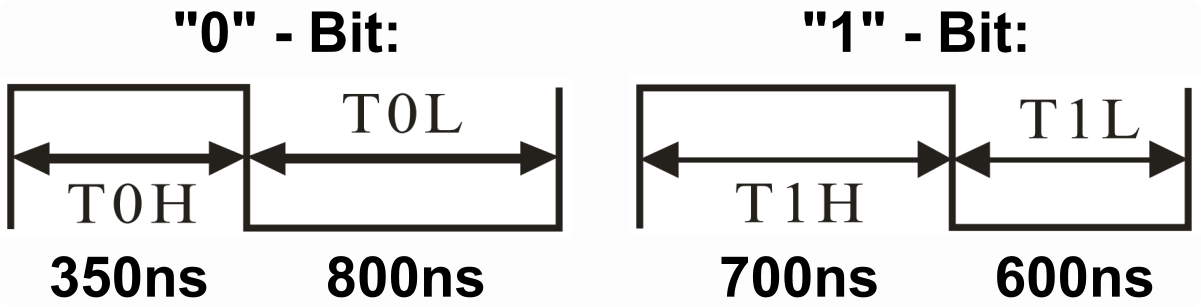
Fortunately, the timing is nowhere near as strict as the data sheet suggests. The following timing rules can be derived from the excellent articles by [Josh Levine](https://wp.josh.com/2014/05/13/ws2812-neopixels-are-not-so-finicky-once-you-get-to-know-them/) and [Tim](https://cpldcpu.wordpress.com/2014/01/14/light_ws2812-library-v2-0-part-i-understanding-the-ws2812/) and should **work with all 800kHz addressable LEDs**:
|Pulse|Parameter|Min|Typical|Max|
|:-|:-|-:|-:|-:|
|T0H|"0"-Bit, HIGH time|65 ns|350 ns|500 ns|
|T0L|"0"-Bit, LOW time|450 ns|800 ns|8500 ns|
|T1H|"1"-Bit, HIGH time|625 ns|700 ns|8500 ns|
|T1L|"1"-Bit, LOW time|450 ns|600 ns|8500 ns|
|TCT|Total Cycle Time|1150 ns|1250 ns|9000 ns|
|RES|Latch, LOW time|9 µs|50 µs|280 µs|
Apart from T0H, the maximum values can be even higher, depending on when the NeoPixels actually latch the sent data (with some types only after 280µs!). This also makes it possible to work **without a buffer** and thus without the use of SRAM, which is the prerequisite for being able to control so many pixels with an ATtiny13. The software essentially only has to ensure that **T0H is a maximum of 500ns and T1H is at least 625ns**, so that the pixels can reliably differentiate "0" from "1" and that the time between sending two bytes is less than the latch time. Assuming that the ATtiny13 runs with a clock frequency of **9.6 MHz**, the following simple bit-banging function for the transmission of a data byte to the NeoPixels string was implemented:
```c
// Send a byte to the pixels string
void NEO_sendByte(uint8_t byte) { // CLK comment
for(uint8_t bit=8; bit; bit--) asm volatile( // 3 8 bits, MSB first
"sbi %[port], %[pin] \n\t" // 2 DATA HIGH
"sbrs %[byte], 7 \n\t" // 1-2 if "1"-bit skip next instruction
"cbi %[port], %[pin] \n\t" // 2 "0"-bit: DATA LOW after 3 cycles
"rjmp .+0 \n\t" // 2 delay 2 cycles
"add %[byte], %[byte] \n\t" // 1 byte MicroCore** and select **ATtiny13**.
- Go to **Tools** and choose the following board options:
- **Clock:** 9.6 MHz internal osc.
- **BOD:** BOD 2.7V
- **Timing:** Micros disabled
- Connect your programmer to your PC and to the ATtiny.
- Go to **Tools -> Programmer** and select your ISP programmer (e.g. [USBasp](https://aliexpress.com/wholesale?SearchText=usbasp)).
- Go to **Tools -> Burn Bootloader** to burn the fuses.
- Open the NeoController sketch and click **Upload**.
### If using the precompiled hex-file
- Make sure you have installed [avrdude](https://learn.adafruit.com/usbtinyisp/avrdude).
- Connect your programmer to your PC and to the ATtiny.
- Open a terminal.
- Navigate to the folder with the hex-file.
- Execute the following command (if necessary replace "usbasp" with the programmer you use):
```
avrdude -c usbasp -p t13 -U lfuse:w:0x3a:m -U hfuse:w:0xff:m -U flash:w:neocontroller.hex
```
### If using the makefile (Linux/Mac)
- Make sure you have installed [avr-gcc toolchain and avrdude](http://maxembedded.com/2015/06/setting-up-avr-gcc-toolchain-on-linux-and-mac-os-x/).
- Connect your programmer to your PC and to the ATtiny.
- Open the makefile and change the programmer if you are not using usbasp.
- Open a terminal.
- Navigate to the folder with the makefile and the sketch.
- Run "make install" to compile, burn the fuses and upload the firmware.
# Operating Instructions
- Connect the NeoPixel-String to respective pin header. Watch the correct pinout!
- Connect a 5V power supply to the USB-C socket.
- The NeoController should immediately start to show a rainbow animation.
- Use your IR remote control to change animation pattern or to switch on/off the NeoPixels.
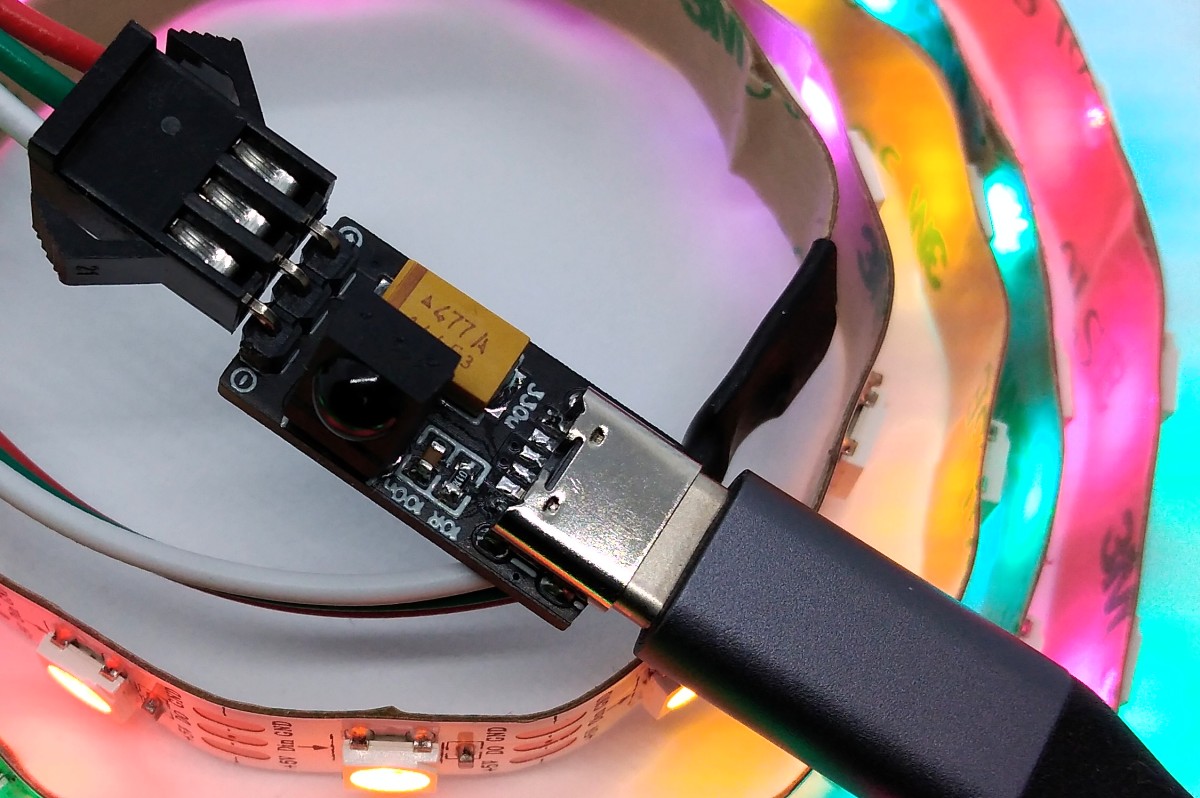
# References, Links and Notes
1. [ATtiny13A datasheet](http://ww1.microchip.com/downloads/en/DeviceDoc/doc8126.pdf)
2. [WS2812 Datasheet](https://cdn-shop.adafruit.com/datasheets/WS2812.pdf)
3. [TSOP4838 Datasheet](https://www.vishay.com/docs/82459/tsop48.pdf)
4. [Josh Levine's Article about NeoPixels](https://wp.josh.com/2014/05/13/ws2812-neopixels-are-not-so-finicky-once-you-get-to-know-them/)
5. [Tim's Article about NeoPixels](https://cpldcpu.wordpress.com/2014/01/14/light_ws2812-library-v2-0-part-i-understanding-the-ws2812/)
6. [AdaFruit NeoPixel Überguide](https://cdn-learn.adafruit.com/downloads/pdf/adafruit-neopixel-uberguide.pdf)
7. [IR Receiver Implementation](https://github.com/wagiminator/ATtiny13-TinyDecoder)
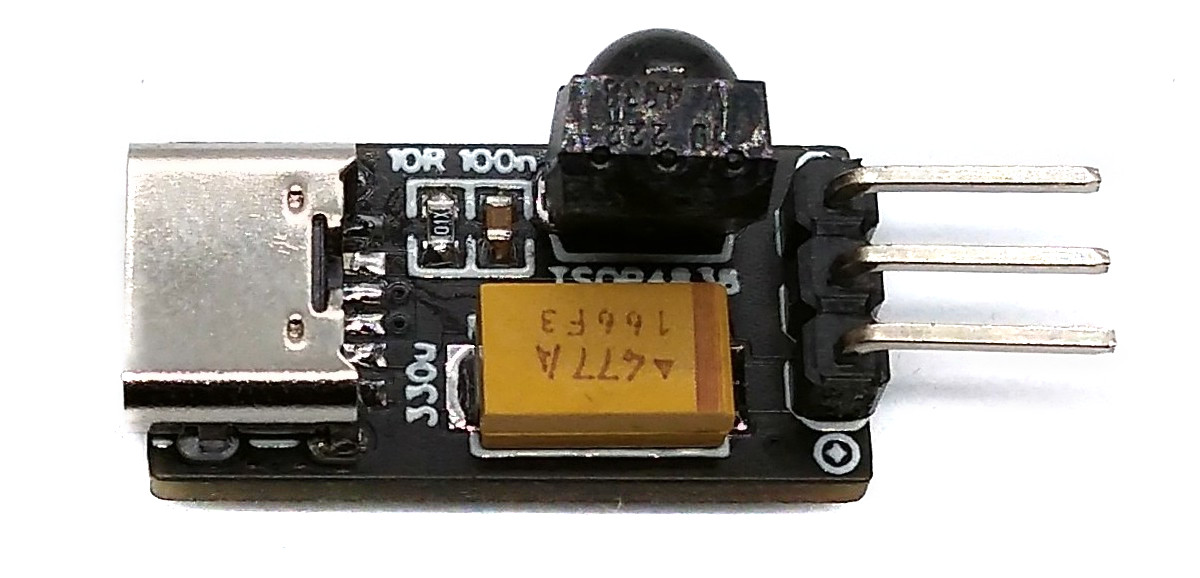
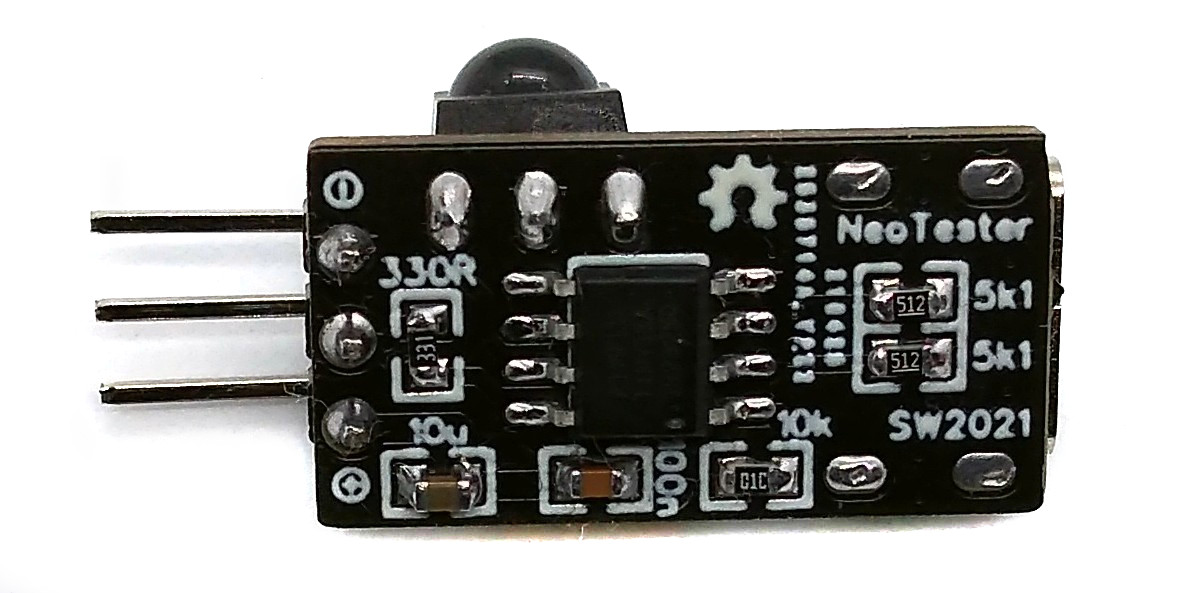
# License

This work is licensed under Creative Commons Attribution-ShareAlike 3.0 Unported License.
(http://creativecommons.org/licenses/by-sa/3.0/)
Forked project will be set private in personal workspace. Do you continue?
Clone
Project
The Pro editor is about to be opened to save as. Do you want to continue?
private message
Send message to wagiminator
Delete
Comment
Are you sure to delete the comment?
Report
ATtiny13 TinyNeoController
Control almost any number of NeoPixels with an IR Remote
Announcer: Stefan Wagner
Creation time: 2021-04-25 09:37:04
Published time:
2022-02-01 04:46:50
*
Report type:
Please select report type
*
Report reason:
Please fill in the reason for your report and the content is 2-1000
words
*
Upload image:
+
Upload image
*
Email address:
Please fill in your email address
Report
*
Report type:
Please select report type
*
Report reason:
Please fill in the reason for your report and the content is 2-1000
words
*
Upload image:
+
Upload image
*
Email address:
Please fill in your email address
Report
Submitted successfully! The review result can be viewed in the personal
center, review notification.
Kind tips
Your EasyEDA usage duration is brief. In order to avoid advertising information, this action is
not supported at present. Please extend your EasyEDA usage duration and try again.
Share
Project
Copy
Copy
Scan the QR code and open it on the mobile terminal