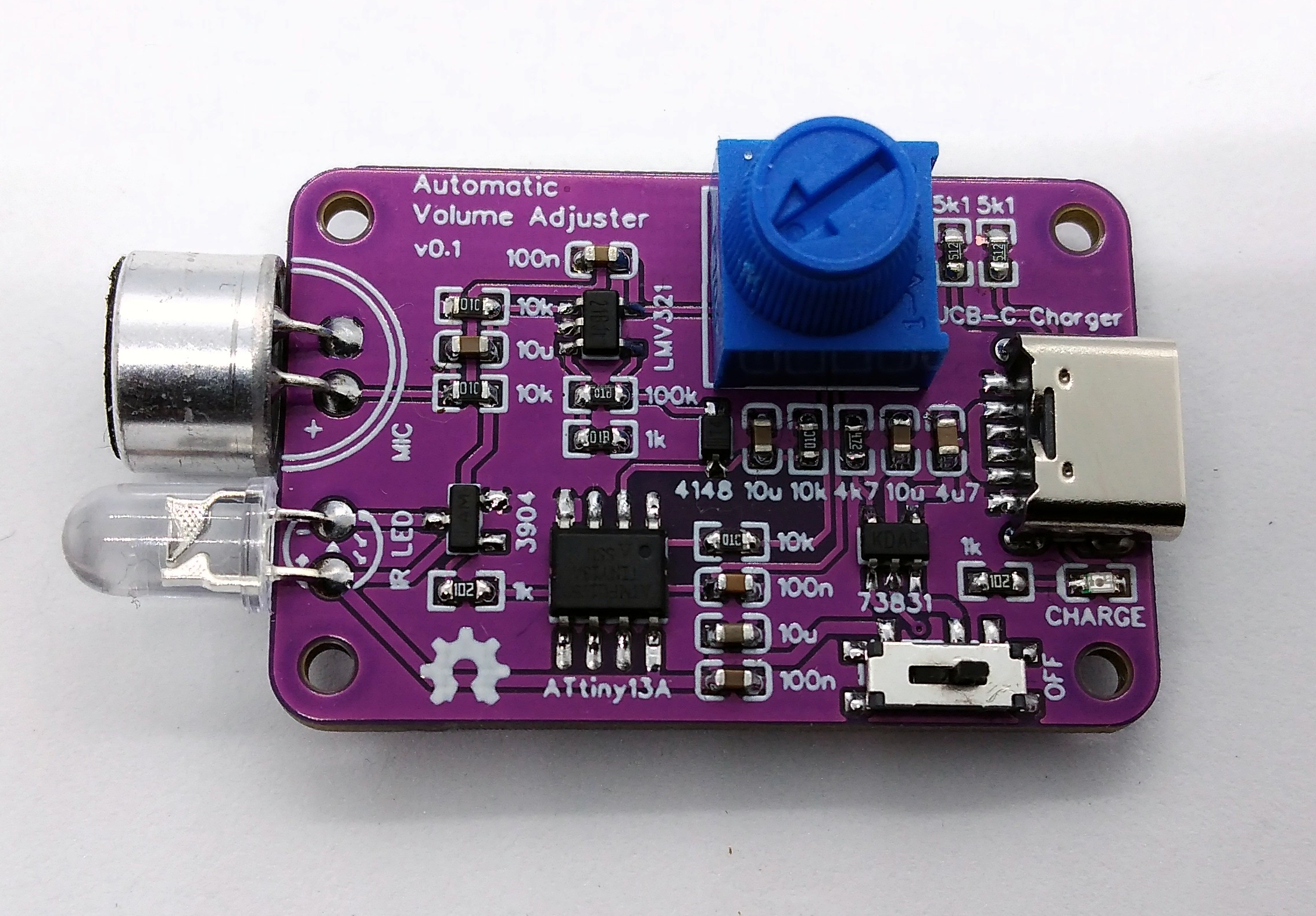
ATtiny13 Volume Adjuster
STDATtiny13 Volume Adjuster
1.7k
0
0
0
Mode:Full
License
:CC-BY-SA 3.0
Creation time:2021-09-27 17:53:25Update time:2021-12-28 13:30:33
Description
Design Drawing
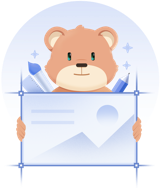
BOM
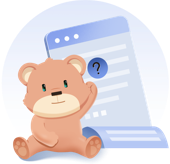

Add to Album
0
0
Share
Report
Project Members
Followers0|Likes0
Related projects
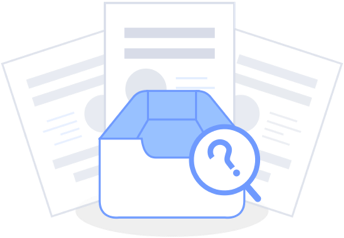
Comment