Your EasyEDA usage duration is brief. In order to avoid advertising information, this action is not supported at present. Please extend your EasyEDA usage duration and try again.
Editor Version×
Standard
1.Easy to use and quick to get started
2.The process supports design scales of 300 devices or 1000 pads
3.Supports simple circuit simulation
4.For students, teachers, creators
Profession
1.Brand new interactions and interfaces
2.Smooth support for design sizes of over 5,000 devices or 10,000 pads
3.More rigorous design constraints, more standardized processes
# Overview
TinyPocketRadio is a simple FM stereo radio based on ATtiny13A and RDA5807MP. It's powered by a CR2032 coin cell battery and can drive 32 Ohm headphones via the 3.5 mm audio plug. The board size is 38 x 23 mm. It has a power switch and three buttons: "Channel+", Volume-" and "Volume+ ".
- Firmware (Github): https://github.com/wagiminator/ATtiny13-TinyPocketRadio
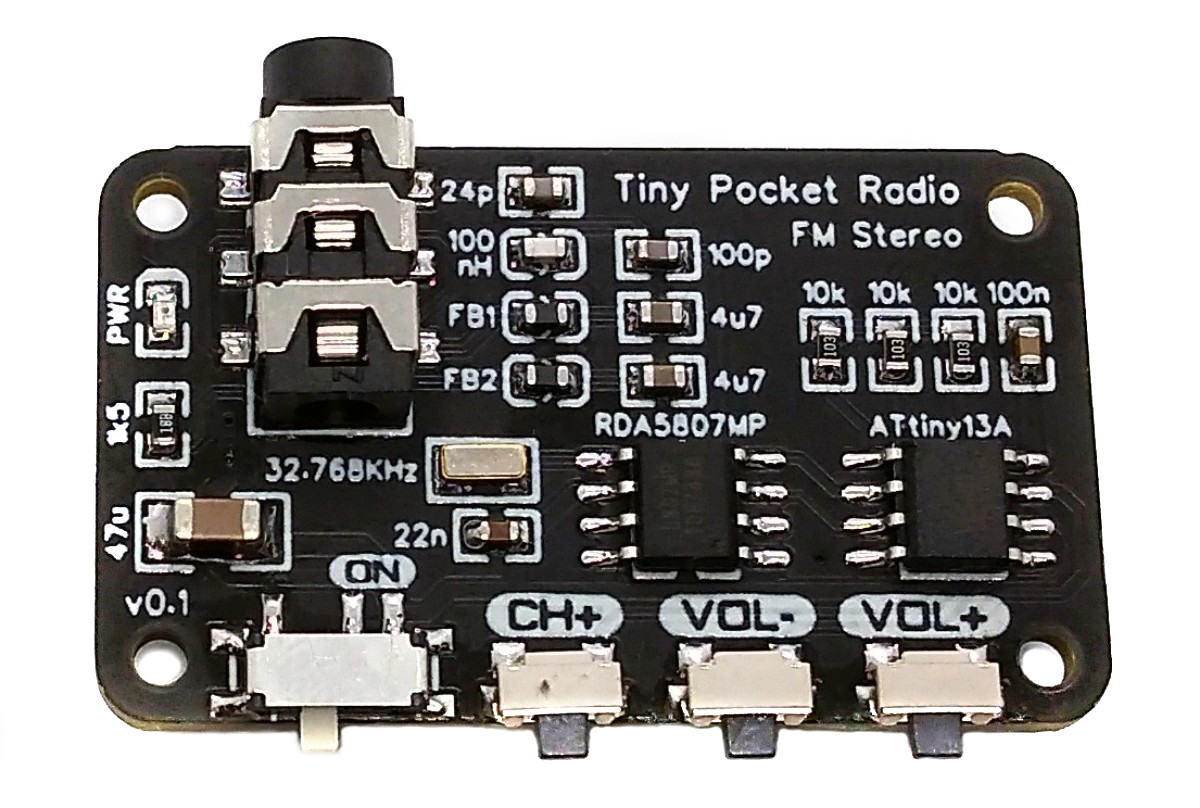
# Hardware
The schematic is shown below:
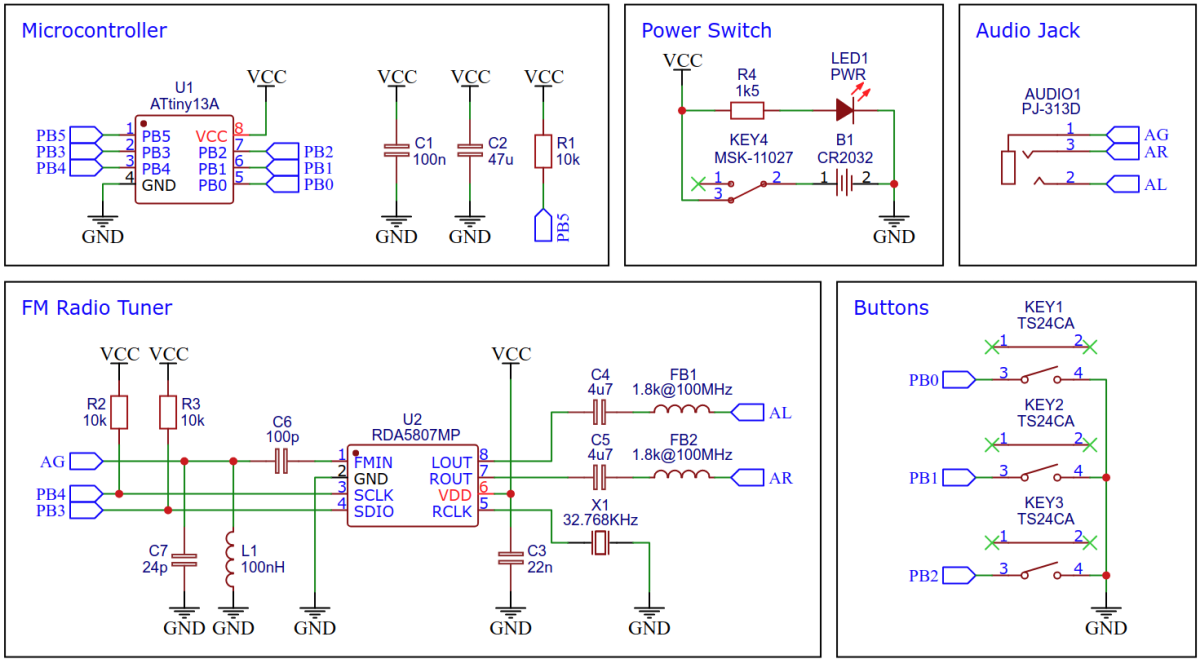
The low-cost RDA5807MP is a single-chip broadcast FM stereo radio tuner with fully integrated synthesizer, IF selectivity, RDS/RBDS and MPX decoder. The tuner uses the CMOS process, support multi-interface and require the least external component. All these make it very suitable for portable devices.
# Software
## I²C Implementation
The I²C protocol implementation is based on a crude bitbanging method. It was specifically designed for the limited resources of ATtiny10 and ATtiny13, but should work with some other AVRs as well. Due to the low clock frequency of the CPU, it does not require any delays for correct timing. In order to save resources, only the basic functionalities which are needed for this application are implemented. For a detailed information on the working principle of the I²C implementation visit [TinyOLEDdemo](https://github.com/wagiminator/attiny13-tinyoleddemo).
## Controlling the RDA5807
The FM tuner IC RDA5807MP is controlled via I²C by the ATtiny. It has six writable 16-bit registers (addresses 0x02 - 0x07) and six readable 16-bit registers (addresses 0x0A - 0x0F). Since no data has to be read from the device for this application, only the writable registers are used. The RDA5807 has two methods of write access, a sequential one in which the registers are always written starting from address 0x02 and an indexed method in which the register address is transferred first and then the content. Both methods are determined by different I²C addresses. To transfer the 16-bit register content, the high byte is sent first. The RDA5807 is controlled by setting or clearing certain bits in the respective registers. The details of the meanings of the individual registers can be found in the data sheet. The current register contents are saved in the RDA_regs array.
```c
// RDA definitions
#define RDA_ADDR_SEQ 0x20 // RDA I2C write address for sequential access
#define RDA_ADDR_INDEX 0x22 // RDA I2C write address for indexed access
#define R2_SEEK_ENABLE 0x0100 // RDA seek enable bit
#define R2_SOFT_RESET 0x0002 // RDA soft reset bit
#define R5_VOLUME 0x000F // RDA volume mask
#define RDA_VOL 5 // start volume
// global variables
uint16_t RDA_regs[6] = {
0b1101001000000101, // RDA register 0x02
0b0001010111000000, // RDA register 0x03
0b0000101000000000, // RDA register 0x04
0b1000100010000000, // RDA register 0x05
0b0000000000000000, // RDA register 0x06
0b0000000000000000 // RDA register 0x07
};
// writes specified register to RDA
void RDA_writeReg(uint8_t reg) {
I2C_start(RDA_ADDR_INDEX); // start I2C for index write to RDA
I2C_write(0x02 + reg); // set the register to write
I2C_write(RDA_regs[reg] >> 8); // send high byte
I2C_write(RDA_regs[reg] & 0xFF); // send low byte
I2C_stop(); // stop I2C
}
// writes all registers to RDA
void RDA_writeAllRegs(void) {
I2C_start(RDA_ADDR_SEQ); // start I2C for sequential write to RDA
for (uint8_t i=0; i> 8); // send high byte
I2C_write(RDA_regs[i] & 0xFF); // send low byte
}
I2C_stop(); // stop I2C
}
// initialize RDA tuner
void RDA_init(void) {
I2C_init(); // init I2C
RDA_regs[0] |= R2_SOFT_RESET; // set soft reset
RDA_regs[3] |= RDA_VOL; // set start volume
RDA_writeAllRegs(); // write all registers
RDA_regs[0] &= 0xFFFD; // clear soft reset
RDA_writeReg(0); // write to register 0x02
}
// set volume
void RDA_setVolume(uint8_t vol) {
RDA_regs[3] &= 0xFFF0; // clear volume bits
RDA_regs[3] |= vol; // set volume
RDA_writeReg(3); // write to register 0x05
}
// seek next channel
void RDA_seekUp(void) {
RDA_regs[0] |= R2_SEEK_ENABLE; // set seek enable bit
RDA_writeReg(0); // write to register 0x02
}
```
## Main Function
The code utilizes the sleep mode power down function to save power. The CPU wakes up on every button press by pin change interrupt, transmits the appropriate command via I²C to the RDA5807 and falls asleep again.
```c
// button pin definitions
#define BT_SEEK PB0
#define BT_VOLM PB1
#define BT_VOLP PB2
#define BT_MASK (1
Forked project will be set private in personal workspace. Do you continue?
Clone
Project
The Pro editor is about to be opened to save as. Do you want to continue?
private message
Send message to wagiminator
Delete
Comment
Are you sure to delete the comment?
Report
ATtiny13 TinyRadio
Simple FM Stereo Pocket Radio for 32 Ohm Headphones and CR2032 Coin Cell Battery
Announcer: Stefan Wagner
Creation time: 2020-11-15 14:56:24
Published time:
2022-01-16 16:40:57
*
Report type:
Please select report type
*
Report reason:
Please fill in the reason for your report and the content is 2-1000
words
*
Upload image:
+
Upload image
*
Email address:
Please fill in your email address
Report
*
Report type:
Please select report type
*
Report reason:
Please fill in the reason for your report and the content is 2-1000
words
*
Upload image:
+
Upload image
*
Email address:
Please fill in your email address
Report
Submitted successfully! The review result can be viewed in the personal
center, review notification.
Kind tips
Your EasyEDA usage duration is brief. In order to avoid advertising information, this action is
not supported at present. Please extend your EasyEDA usage duration and try again.
Share
Project
Copy
Copy
Scan the QR code and open it on the mobile terminal